Connect
In a nutshell
Integrate APIs for managing sub-merchants and flow of funds in your SaaS platform or Marketplace.
Introduction
Certain businesses provide platforms that connect sub-merchants with buyers for seamless transactions. These businesses handle many complex and resource intensive operational tasks, such as:
- Onboarding sellers and service providers
- Splitting transactions between multiple parties
- Reconciliation of funds
- Chargebacks, disputes, and fraud management
Paystack Connect simplifies operational complexities for these businesses, allowing them to focus on delivering value to their users. With our APIs, businesses can onboard their sub-merchants and manage flow of funds without a hassle.
As you read through this page, you might need to familiarise yourself with some of the terms used, as described in the table below:
Term | Meaning |
---|---|
Platform | This is a business entity that manages sellers and service providers with the aim of connecting them with buyers. |
Sub-merchant | This is a business entity that provides goods and/or services to buyers. |
SaaS | Software as a Service is a business model that allow companies use software to provide services to their customers via the internet. |
Marketplace | This is the physical or virtual location where vendors meet to sell goods or services to customers. |
Onboarding and KYC | This is the process of verifying the details of your customer in order to confirm that they are who they say they are. |
Fraud and dispute risk | This is the financial risk (i.e. losses) due to fraud or disputes initiated by a buyer. |
Integration flow
Getting started with the Connect APIs involves 3 steps:
- Onboard the sub-merchant
- Accept payment
- Manage post-payment processes
Platform integration prerequisite
You need to have a Paystack account before proceeding. If you don’t have an account you can create one via the dashboard or follow the instruction in our support article.
To onboard a sub-merchant, you need to create an account on your platform and link the account to the sub-merchant’s business. Before creating an account you need to make a decision on two factors:
- Risk bearer: On completion of a transaction, the customer can raise a dispute or request a refund. These are known as transaction risks. You need to decide if you will be bearing the risk or not. You can check out the Managing Post-payment Processes section to learn more about managing transaction risks.
- KYC requirement: When onboarding sub-merchants, you might need to verify their details to confirm their risk profile. You can either use your KYC verification flow or delegate it to Paystack.
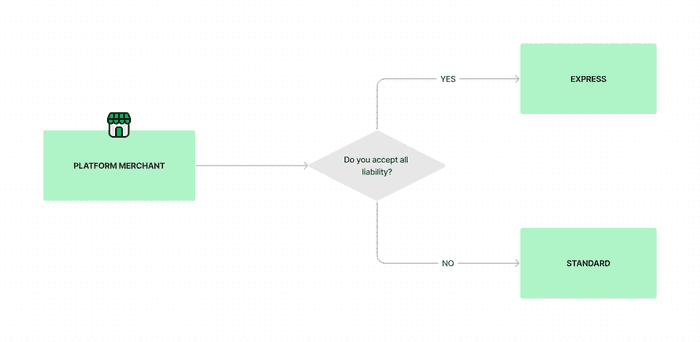
There are two integration flows based on these factors:
- Standard flow
- Express flow
Standard flow
With the standard flow, the sub-merchant onboards directly on Paystack, so Paystack handles the KYC process and the sub-merchant bears the transaction risk. The sub-merchant has access to Paystack dashboard and APIs to view their transactions, manage chargebacks, fraud and reconciliation. When a platform is connected to the sub-merchant’s Paystack account, the platform can transact on behalf of the sub-merchant, with limited visibility into their operations.
Express flow
In this flow, the platform onboards the sub-merchant and bears the transaction risk. The platform would transact on their Paystack account and split payments with the sub-merchant, so during onboarding, the platform needs to collect the sub-merchant’s bank details.
The table below summarises the distinct features of each integration flow:
Feature | Standard | Express |
---|---|---|
Onboarding and KYC | Paystack onboards sub-merchant. | Platform onboards sub-merchant. |
Flow of funds | Charges are created directly in the sub-merchants Paystack account. Platform has some control over fee disbursement. | The platform can control the split, route and payout sub-merchant’s funds. |
Fraud and dispute liability | The sub-merchant bears the transaction risks. | The platform bears the transaction risks. |
UI customisation | Default Paystack branded UI. | Platform creates their UI with their brand colours and logo. |
Development effort | Lowest effort. | Medium effort. |
Revenue opportunity | Paystack set pricing with sub-merchants, the platform can apply service fees, and volume-based revenue share. | The platform gets a wholesale rate from Paystack with controls over pricing and the transaction fee split. |
Sub-merchant support | Paystack. | Platform. |
Create an account
When you’ve determined the requirements for the sub-merchant and the integration flow, you can make a POST
request to the create accountAPI endpoint:
1#!/bin/sh2curl https://api.paystack.co/connect/accounts3-H "Authorization: Bearer YOUR_SECRET_KEY"4-H "Content-Type: application/json"5-d '{6 "business_name": "Boom Boom Lite",7 "currency": "NGN",8 "emails": ["tope@demo.com"],9 "split_type": "percentage",10 "platform_share": 1,11 "own_risk": false,12 "require_onboarding": true13 }'14-X POST
1{2 "message": "Account created",3 "data": {4 "account_id": "C_ACT_4KRPYCAACP",5 "business_name": "Boom Boom Lite",6 "platform_integration_id": 463433,7 "platform_slug": "test-boom-boom-ng",8 "platform_id": "US2VSIMVAB",9 "domain": "test",10 "submerchant_integration_id": null,11 "status": "created",12 "currency": "NGN",13 "emails": [14 "tope@demo.com"15 ],16 "split_type": "percentage",17 "platform_share": 1,18 "setup_link": "https://connect.paystack.co/platform_name/onboarding?token=eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhY2NvdW50SWQiOiJDX0FDVF80S1JQWUNBQUNQIiwicGxhdGZvcm1JZCI6IlVTMlZTSU1WQUIiLCJpYXQiOjE3MTEwMjY1MTUsImV4cCI6MTcxMTYzMTMxNX0.ttR3Zv8Pw00n6tkeIU42eOrt5aH_aWk2e4mWEDhIy1A"19 }20}
Among other details, the response contains an account_id
and a setup_link
. The account_id
is a unique identifier for the created account. You should save the account_id
against the sub-merchant details in your database.
The setup_link
is to be used by your sub-merchant to link their account to complete the onboarding to your platform. We send an email, containing this link, to the sub-merchant.
Accept payment
When you’ve successfully onboarded your sub-merchant, you can begin to carry out transactions on their behalf. To do this, you can make a POST
request to the Initialize TransactionAPI endpoint, adding the sub-merchants account ID in the X-Connect-Account
header of your request:
1#!/bin/sh2curl https://api.paystack.co/transaction/initialize3-H "Authorization: Bearer YOUR_SECRET_KEY"4-H "X-Connect-Account: C_ACT_KI6CZ27HUZ"5-H "Content-Type: application/json"6-d '{7 "email": "customer@demo.com",8 "amount": 500000,9 "connect_split": [10 {11 "account_id": "C_ACT_PBMKJ77OY3",12 "share": 1013 },14 {15 "account_id": "C_ACT_KI6CZ27HUZ",16 "share": 6017 }18 ]19 }'20-X POST
1{2 "status": true,3 "message": "Authorization URL created",4 "data": {5 "authorization_url": "https://checkout.paystack.com/nkdks46nymizns7",6 "access_code": "nkdks46nymizns7",7 "reference": "nms6uvr1pl"8 }9}
At the point of initializing a transaction, you might decide to split payment with another sub-merchant. To do this, you can pass the connect_split
array in the request body. There are two things to note when overriding the default split configuration:
- The connect account specified in the header must also be added in the
connect_split
array - The share of each sub-merchant added is deducted from the platform’s share
The data
object in the response contains an authorization_url
that you can redirect the customer to complete the payment. On successful payment, we send a charge.success
event to your webhook URL.
1{2 "event": "charge.success",3 "data": {}4}
If you don’t have a webhook URL, you can check out the webhooks page to learn how to set it up.
Managing post-payment processes
Sometimes, customers may file complaints after making payment for goods and service. Other times, you need to ensure you books are up to date. All these are known as post-payment processes. It is important you are aware of how to manage them in other to ensure you have a seamless business operation.
Disputes and chargebacks
A dispute is a complaint made by a customer to their bank if they are unsatisfied with a transaction. Common reasons for disputes include not receiving an expected product, discrepancies between anticipated and actual products, unauthorized charges, and unrecognized account activity.
A chargeback is a request for the reversal of a payment made to your business by the customer. The customer makes this request through their bank.
They both require you to review the customer’s claim and take an action. You can manage disputes and chargebacks from your Paystack Dashboard or you can automate the process via API. You can learn more about Disputes and chargebacks from our support articles.
Reconciliation
Reconciliation involves confirming that the product sold or service rendered matches the amount received. This is to enable you keep track of your revenue. You can view and manage your transactions via the dashboard or using the List TransactionAPI and SettlementsAPI endpoints. You can check out our support articles for a deep dive on how transactions and settlement works.